Introduction
The Event interface represents an interaction with the DOM, such as clicks, key presses, and mouse movements. Two of its key properties, event.target
and event.currentTarget
, are often misunderstood. In this post, we’ll dive into their roles and differences, using practical examples to demonstrate their behavior.
Understanding event.target
and event.currentTarget
What is event.target
?
event.target refers to the element that triggered the event. This is the original source of the event, where the interaction (e.g., a click) first occurred.
What is event.currentTarget
?
event.currentTarget
refers to the element to which the event handler is attached, regardless of whether it was the original trigger. This is particularly important when handling events that bubble up through the DOM.
Examples
Example of event.target
Let’s explore how event.target
works. Consider the following HTML structure:
<body>
<form>
FORM
<div>
DIV
<p>P</p>
</div>
</form>
<script>
const form = document.querySelector("form");
const div = document.querySelector("div");
const p = document.querySelector("p");
form.onclick = function (event) {
console.log("form: ", event.target);
};
div.onclick = function (event) {
console.log("div: ", event.target);
};
p.onclick = function (event) {
console.log("p: ", event.target);
};
</script>
</body>
The code renders this:

In this example, we have event listeners for clicks on the form
, div
, and p
elements. Here’s what happens when we click the p
tag:
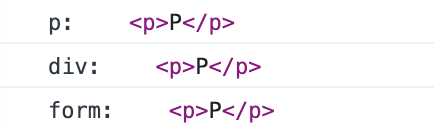
Even though we clicked on the p
element, the div
and form
elements also logged the event due to event bubbling. However, all three logs show p
as the event.target
.
Key takeaway: Event bubbling does not affect the event.target
. Regardless of how far the event bubbles, event.target remains the element that initiated the event.
Clicking div
and form
results the following respectively:
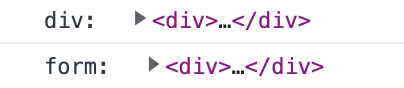

Example of event.currentTarget
Now, let’s replace event.target
with event.currentTarget
in the previous example:
form.onclick = function (event) {
console.log("form: ", event.currentTarget);
};
div.onclick = function (event) {
console.log("div: ", event.currentTarget);
};
p.onclick = function (event) {
console.log("p: ", event.currentTarget);
};
When you click the p
element now, the logs show the element that owns each event handler as the event.currentTarget
.
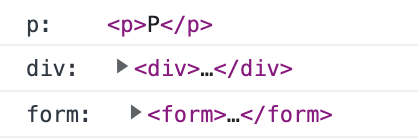
Clicking div
and form
results the following respectively:
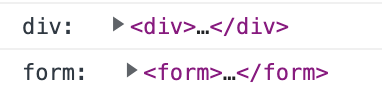

For an element to be the event.currentTarget
, it must have an event handler for the specified event. If an element has a handler for a different event, it won’t qualify as the event.currentTarget
for the current event. Here’s an example where we change the p
element’s handler to listen for ondrop
instead of onclick
:
...
p.ondrop = function(event) {
console.log("p: ", event.currentTarget)
}
Now, clicking on p won’t invoke the handler:
Now, clicking p
again and we can see that handler for p
is NOT invoked because the handler only handles drop events and not click events.
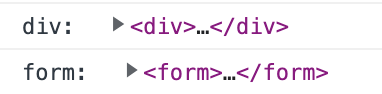
Conclusion
In summary:
event.target
is always the element that triggered the event. It remains constant as the event bubbles up.event.currentTarget
refers to the element where the event handler is attached. It changes as the event bubbles and reaches new elements, provided they have an appropriate handler for that event.
Understanding these two properties will help you manage event handling more effectively, especially when dealing with complex DOM structures and event propagation.